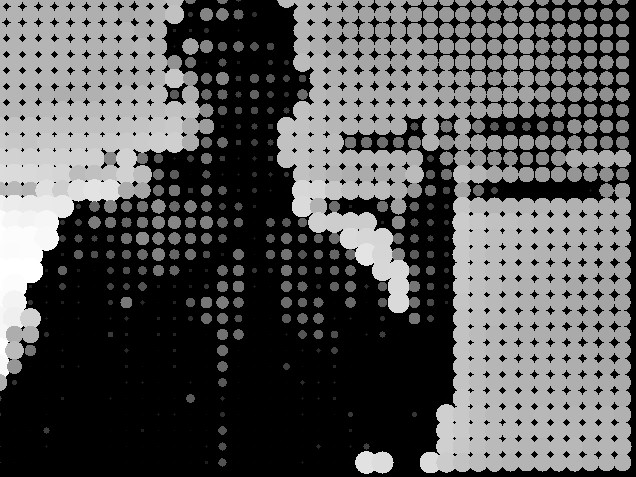
import processing.video.*;
Capture video;
void setup() {
size(640, 480);
// Initialize the video at 40x30 resolution
video = new Capture(this, 40, 30,30);
// Start capturing the images from the camera
video.start();
noStroke();
noSmooth();
}
void captureEvent(Capture c) {
c.read();
}
void draw() {
background(0); // Clear the screen before drawing new frame
// Check if video is available to prevent errors
if (video.available()) {
video.read();
}
// Load the video pixels
video.loadPixels();
// Calculate scaling factor to fit the low-resolution video on the canvas
float scaleFactorX = (float) width / video.width;
float scaleFactorY = (float) height / video.height;
// Iterate through each pixel in the video
for (int y = 0; y < video.height; y++) {
for (int x = 0; x < video.width; x++) {
int index = x + y * video.width;
int pixelColor = video.pixels[index];
// Extract the RGB components
int r = (pixelColor >> 16) & 0xff;
int g = (pixelColor >> 8) & 0xff;
int b = pixelColor & 0xff;
// Calculate the brightness
float brightness = (r + g + b) / 3.0;
// Map the brightness to a circle size
//loat circleSize = map(brightness, 0, 255, 0, brightness);
// Set the fill color to the original pixel color
fill(brightness);
// Draw the circle at the corresponding position
circle(x * scaleFactorX, y * scaleFactorY, brightness*.1);
}
}
// Display the video feed on the canvas
//set(0, 0, video);
}
MIRROR
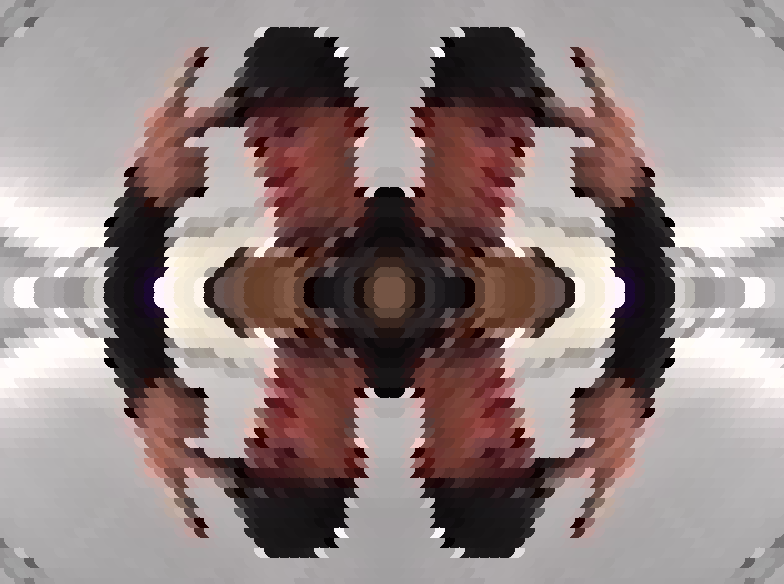
import processing.video.*;
Capture video; // create camera instance or object
void setup() {
size(800, 600);
// Initialize the video at 40x30 resolution
video = new Capture(this, 80, 60,30);
// Start capturing the images from the camera
video.start();
noStroke();
noSmooth();
}
void captureEvent(Capture c) {
c.read();
}
void draw() {
background(0); // Clear the screen before drawing new frame
// Load the video pixels
video.loadPixels();
// Iterate through each pixel in the video
for (int y = 0; y < video.height/2; y++) {
for (int x = 0; x < video.width/2; x++) {
int index = x + y * video.width;
int pixelColor = video.pixels[index];
// Extract the RGB components
int r = (pixelColor >> 16) & 0xff;
int g = (pixelColor >> 8) & 0xff;
int b = pixelColor & 0xff;
// Calculate the brightness
float brightness = (r + g + b) / 3.0;
// Map the brightness to a circle size
//loat circleSize = map(brightness, 0, 255, 0, brightness);
//if(brightness>180){
// Set the fill color to the original pixel color
fill(pixelColor);
// Draw the circle at the corresponding position
circle(x * 10, y * 10, 22); // LO
circle(width- x * 10 -10, y * 10,22); // RO
circle(x * 10, height-y*10 - 10, 22); // LU
circle(width -x * 10 -10, height-y*10 - 10, 22); // LU
//}
}
}
// Display the video feed on the canvas
// set(0, 0, video);
}
ASCII BASIC
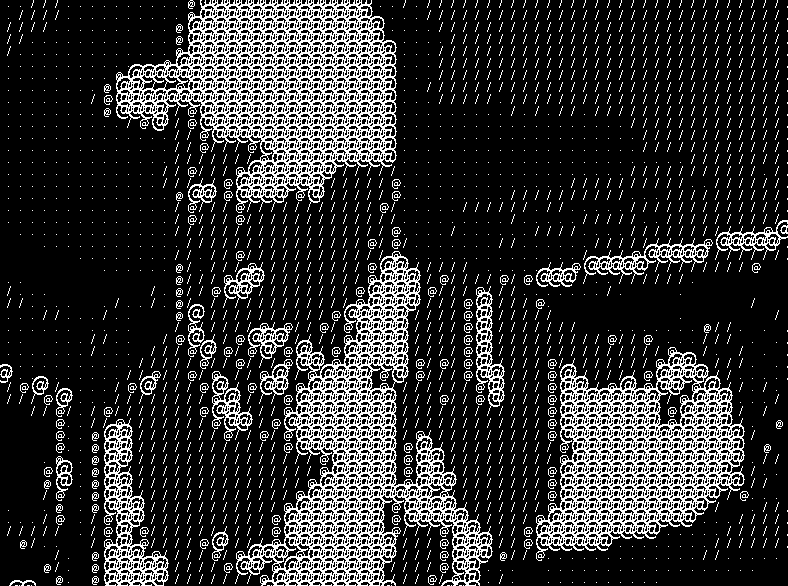
import processing.video.*;
Capture video; // create camera instance or object
void setup() {
size(800, 600);
// Initialize the video at 40x30 resolution
video = new Capture(this, 80, 60,30);
// Start capturing the images from the camera
video.start();
noStroke();
noSmooth();
}
void captureEvent(Capture c) {
c.read();
}
void draw() {
background(0); // Clear the screen before drawing new frame
// Load the video pixels
video.loadPixels();
// Iterate through each pixel in the video
for (int y = 0; y < video.height; y++) {
for (int x = 0; x < video.width; x++) {
int index = x + y * video.width;
int pixelColor = video.pixels[index];
// Extract the RGB components
int r = (pixelColor >> 16) & 0xff;
int g = (pixelColor >> 8) & 0xff;
int b = pixelColor & 0xff;
// Calculate the brightness
float brightness = (r + g + b) / 3.0;
//fill(255);
// text(round(brightness), x*22, y*22);
if(brightness<80){
fill(255);
text("@", x*12,y*12);
textSize(22);
}else if( brightness>=80 && brightness<170 ) {
textSize(12);
text("/", x*12,y*12);
}else if( brightness>=170 && brightness<240 ) {
textSize(10);
text(".", x*12,y*12);
}
}
}
// Display the video feed on the canvas
// set(0, 0, video);
}
the wobbly fluffy me spikes
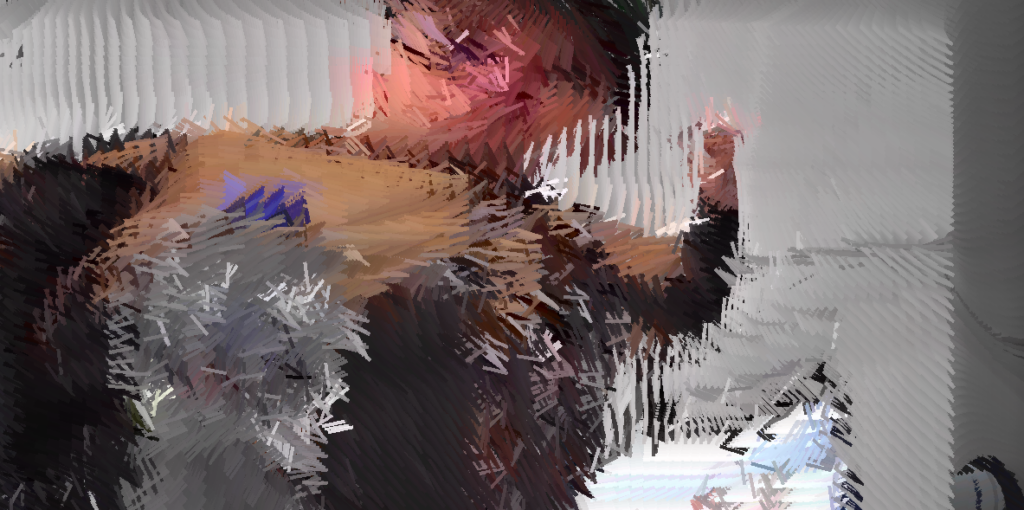
import processing.video.*;
PFont myfont;
Capture video; // create camera instance or object
void setup() {
size(1200, 600);
//fullScreen();
// Initialize the video at 40x30 resolution
video = new Capture(this, 80, 60,30);
// Start capturing the images from the camera
video.start();
noStroke();
noSmooth();
myfont = loadFont("FreestyleScript-Regular-30.vlw");
textFont(myfont);
}
void captureEvent(Capture c) {
c.read();
}
void draw() {
fill(0,4);
rect(0,0,width,height);
// Load the video pixels
video.loadPixels();
// calculate a width/ height ratio to place each pixel canvas wide
float wb = width/video.width;
float hb = height/video.height;
// Iterate through each pixel in the video
for (int y = 0; y < video.height; y++) {
for (int x = 0; x < video.width; x++) {
int index = x + y * video.width;
int pixelColor = video.pixels[index];
// Extract the RGB components
int r = (pixelColor >> 16) & 0xff;
int g = (pixelColor >> 8) & 0xff;
int b = pixelColor & 0xff;
// Calculate the brightness
float brightness = (r + g + b) * .33;
fill(pixelColor,222);
float a = map( brightness, 0 , 255, 0, PI*2 );
// duplicate pixel position
float xpos = x;
float ypos = y;
xpos += sin( xpos*.1 + millis()*.001 )*6;
ypos += sin( ypos*.1 + millis()*.0014 )*6;
pushMatrix();
translate(xpos*wb, ypos*hb);
rotate( a );
rect( 0,0, 30,4 );
popMatrix();
}
}
// Display the video feed on the canvas
// set(0, 0, video);
}
SLITSCAN
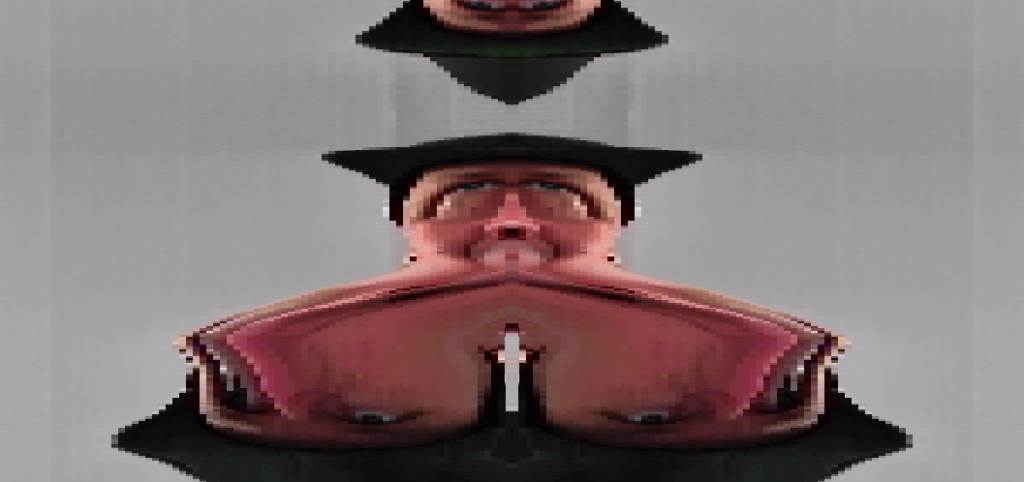
import processing.video.*;
PFont myfont;
Capture video; // create camera instance or object
void setup() {
size(1200, 600);
//fullScreen();
// Initialize the video at 40x30 resolution
video = new Capture(this, 160, 120,30);
// Start capturing the images from the camera
video.start();
noStroke();
noSmooth();
}
void captureEvent(Capture c) {
c.read();
}
float yscan = 0;
void draw() {
yscan += 1;
if(yscan>height){
yscan = 0;
}
/*
fill(0,4);
rect(0,0,width,height);
*/
// Load the video pixels
video.loadPixels();
// calculate a width/ height ratio to place each pixel canvas wide
float wb = width/video.width;
float hb = height/video.height;
// Iterate through each pixel in the video
for (int y = 20; y < 21; y++) {
for (int x = 0; x < video.width/2; x++) {
int index = x + y * video.width;
int pixelColor = video.pixels[index];
// Extract the RGB components
int r = (pixelColor >> 16) & 0xff;
int g = (pixelColor >> 8) & 0xff;
int b = pixelColor & 0xff;
// Calculate the brightness
float brightness = (r + g + b) * .33;
fill(pixelColor);
rect( x*wb, yscan, wb, 10 );
rect( width-x*wb-100, yscan, wb, 10 );
}
}
// Display the video feed on the canvas
// set(0, 0, video);
}