basic interaction scene
Based on the previous setup, this sketch applies basic key interaction to „navigate“ through a image made scene. This is more of an illusion, than an ai generated room. But who cares 😉
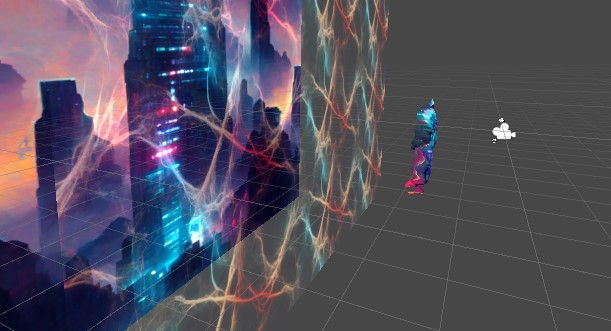
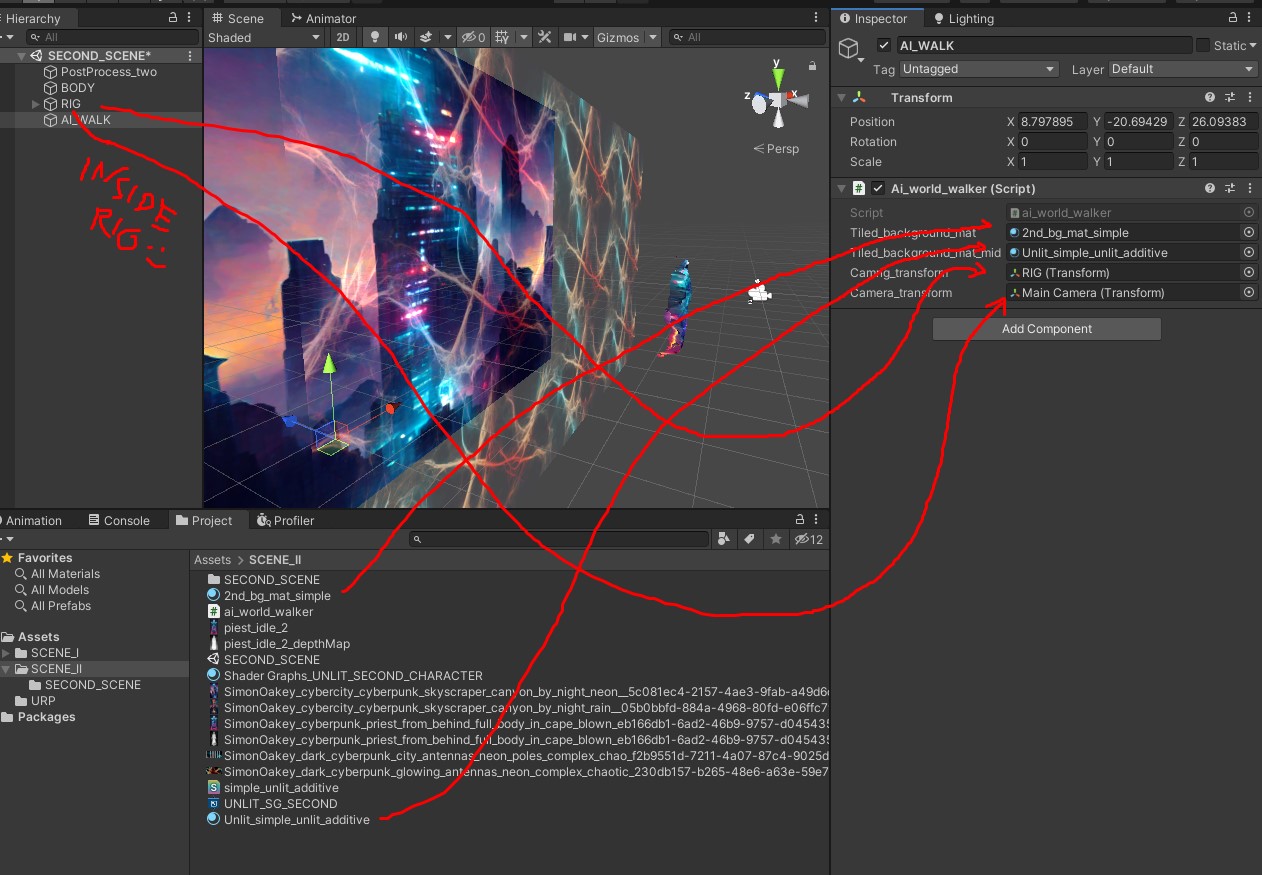
ai_world_walker.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class ai_world_walker : MonoBehaviour { // two separate materials for each parallax layer public Material tiled_background_mat; public Material tiled_background_mat_mid; // offset vectors - pretty clear Vector2 _off = Vector2.zero; Vector2 _rig_rot_off = Vector3.zero; Vector3 idle_motion_off = Vector3.zero; // all transform data of the camera rig and the camera itself public Transform camrig_transform; public Transform camera_transform; // speed of motion and rotation - you can play with this float speed = 10.0f; float rotationSpeed = 30.0f; void Update() { // save time in t var - because typing Time.time sucks float t = Time.time; // slow idle motion of the rig idle_motion_off.x = Mathf.Sin(t*.13f)*.4f; idle_motion_off.y = Mathf.Sin(t*.1f)*.15f; idle_motion_off.z = Mathf.Sin(t*.16f)*.13f; // get key input from player one float translation = Input.GetAxis("Vertical") * speed; float rotation = Input.GetAxis("Horizontal") * rotationSpeed * .09f; // apply input to texture offset vector _off.y = translation*.02f; _off.x += rotation*.004f; // modify offset of map! // apply offset vector to both materials tiled_background_mat.SetTextureOffset("_BaseMap", _off); tiled_background_mat_mid.SetTextureOffset("_BaseMap", _off); // rotate and move the rig too - makes it more dynamic _rig_rot_off.y = -rotation*1.63f; camrig_transform.rotation = Quaternion.Slerp(camrig_transform.rotation,Quaternion.Euler(_rig_rot_off), .00914f); camrig_transform.position = idle_motion_off; // make the camera breathe motion along y axis camera_transform.localPosition = new Vector3(Mathf.Sin(t*.6f)*.3f, Mathf.Sin(t*1.6f)*.1f,-10f) ; // let the camera zoom in with user input Camera.main.fieldOfView = 55f -translation*1f ; } }
basic flythrough scene
This simple shadergraph example displaces a dense vertex mesh along a grayscale depthmap in z-dimension. The depthmap is generated by a pretty powerful free online tool available at https://convert.leiapix.com/#/.
Having the #midjourney generated figurine and a background image, that is seamless ( use –tile prompt addon ) and a proper depthmap. we can construct a simple scene.
files
The complete project – part ONE & TWO – is temporarily over here: ( UNITY 2019.4.32f LTS)
https://box.burg-halle.de/s/bqn37b8bk2eKZ4J