Ähnliche Beiträge: Keine ähnlichen Artikel gefunden.
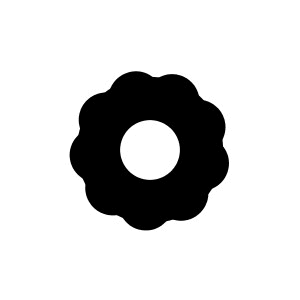
// Initialize a variable to keep track of time
int t = 0;
void setup() {
// Set the size of the canvas to 300x300 pixels
size(300, 300);
// Set the frame rate to 20 frames per second
frameRate(20);
// Disable drawing the outline of shapes
noStroke();
}
void draw() {
// Set the background color to white
background(255);
// Update the time variable to the current frame count
t = frameCount;
// Set the fill color to black
fill(0);
// Save the current transformation matrix
pushMatrix();
// Move the origin to the center of the canvas
translate(width * 0.5, height * 0.5);
// Scale the entire drawing by a factor that varies over time
scale(1.5 + sin(t * 0.1) * 0.2);
// Draw a large circle at the center
circle(0, 0, 88);
// Loop to draw 9 smaller circles around the large circle
for (int i = 0; i < 9; i++) {
// Save the current transformation matrix
pushMatrix();
// Set the fill color to black
fill(0);
// Rotate the drawing by a varying angle
rotate(t * 0.01 + i * (TWO_PI / 9));
// Move the origin to a point on the circle's perimeter
translate(32, 22 + sin(t * 0.2) * 22);
// Draw a smaller circle at the new origin
circle(0, 0, 33);
// Restore the previous transformation matrix
popMatrix();
}
// Restore the previous transformation matrix
popMatrix();
// Set the fill color to white
fill(255);
// Save the current transformation matrix
pushMatrix();
// Move the origin to the center of the canvas
translate(width * 0.5, height * 0.5);
// Scale the drawing by a smaller factor that varies over time
scale(0.9 + sin(t * 0.3) * 0.2);
// Draw another circle at the center, inside the large circle
circle(0, 0, 62);
// Restore the previous transformation matrix
popMatrix();
// Check if the mouse is pressed
if (mousePressed) {
// Save the current frame as an image file
saveFrame("out/f_###.jpg");
}
}