FULL SETUP
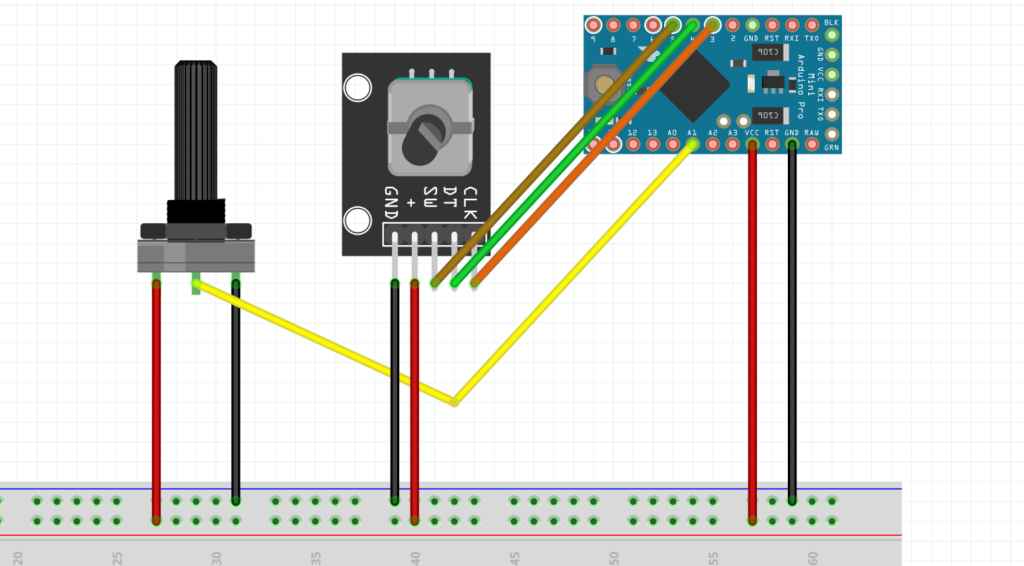
#define encoderPinA 3 // Define pin A for the encoder #define encoderPinB 4 // Define pin B for the encoder #define encoderSW 5 // Define pin SW for the encoder (optional) #define poti_pin A1 int poti_val = 0; volatile int encoderValue = 0; // Variable to store the dial value volatile int lastEncoded = 0; // Variable to store the last encoded value void setup() { Serial.begin(9600); // Initialize serial communication at 9600 baud pinMode(encoderPinA, INPUT); // Set encoder A pin as input pinMode(encoderPinB, INPUT); // Set encoder B pin as input pinMode(encoderSW, INPUT_PULLUP); // Set encoder SW pin as input with pull-up resistor (optional) // Attach interrupts to the encoder pins attachInterrupt(digitalPinToInterrupt(encoderPinA), updateEncoder, CHANGE); attachInterrupt(digitalPinToInterrupt(encoderPinB), updateEncoder, CHANGE); Serial.println("Encoder Test:"); } void loop() { // Print the encoder value static int lastPrintValue = -1; if (encoderValue != lastPrintValue) { Serial.print("Encoder Value: "); Serial.println(encoderValue); lastPrintValue = encoderValue; } // Check if the encoder button is pressed (optional) if (digitalRead(encoderSW) == LOW) { // read out poti value poti_val = analogRead( poti_pin ); Serial.println("Button Pressed with poti value: "); Serial.print (poti_val); delay(200); // Debounce delay } } void updateEncoder() { int MSB = digitalRead(encoderPinA); // Read the MSB (most significant bit) int LSB = digitalRead(encoderPinB); // Read the LSB (least significant bit) int encoded = (MSB << 1) | LSB; // Combine the two bits to get the encoded value int sum = (lastEncoded << 2) | encoded; // Shift last encoded value and combine with current if (sum == 0b1101 || sum == 0b0100 || sum == 0b0010 || sum == 0b1011) encoderValue++; if (sum == 0b1110 || sum == 0b0111 || sum == 0b0001 || sum == 0b1000) encoderValue--; lastEncoded = encoded; // Store the current encoded value for the next change }
LDR
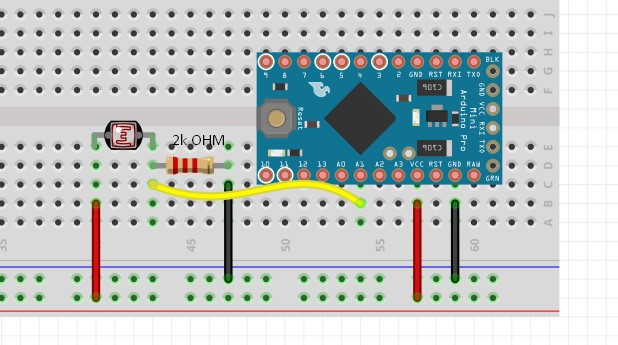
#define LDRPin A1 // Define the pin where the LDR is connected void setup() { Serial.begin(9600); // Initialize serial communication at 9600 baud pinMode(LDRPin, INPUT); // Set the LDR pin as an input } void loop() { int ldrValue = analogRead(LDRPin); // Read the analog value from the LDR Serial.print("LDR Value: "); // Print the label Serial.println(ldrValue); // Print the LDR value delay(500); // Wait for half a second before the next reading }
distance
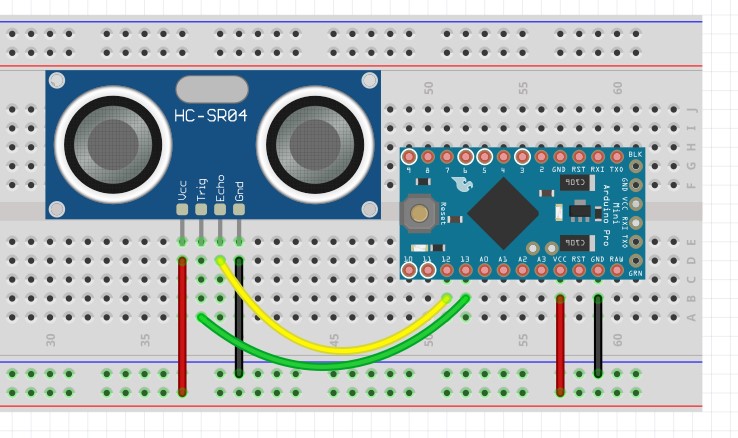
#define trigPin 13 #define echoPin 12 long duration, distance; float voltage, speedOfSound = 0.0343; // Speed of sound in cm/us void setup() { Serial.begin(9600); pinMode(trigPin, OUTPUT); pinMode(echoPin, INPUT); } void loop() { digitalWrite(trigPin, LOW); delayMicroseconds(2); digitalWrite(trigPin, HIGH); delayMicroseconds(10); // Added this line digitalWrite(trigPin, LOW); duration = pulseIn(echoPin, HIGH); distance = (duration / 2.0) * speedOfSound; // includes Speed of Sound conversion factor into centimeters and microseconds Serial.println(distance); delayMicroseconds(100); // wait }
pir sensor
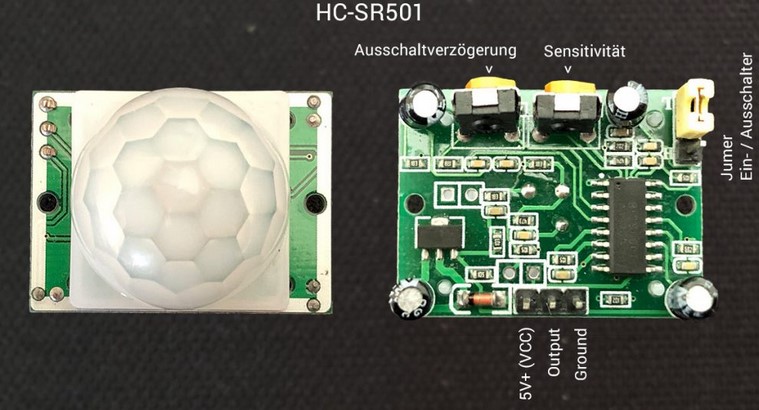
IR distance interruptor
https://wiki.seeedstudio.com/Grove-IR_Distance_Interrupter_v1.2/
void setup() { Serial.begin(9600); pinMode(6,INPUT); } void loop() { while(1) { delay(500); if(digitalRead(6)==LOW) { Serial.println("Somebody is here."); } else { Serial.println("Nobody."); } } }