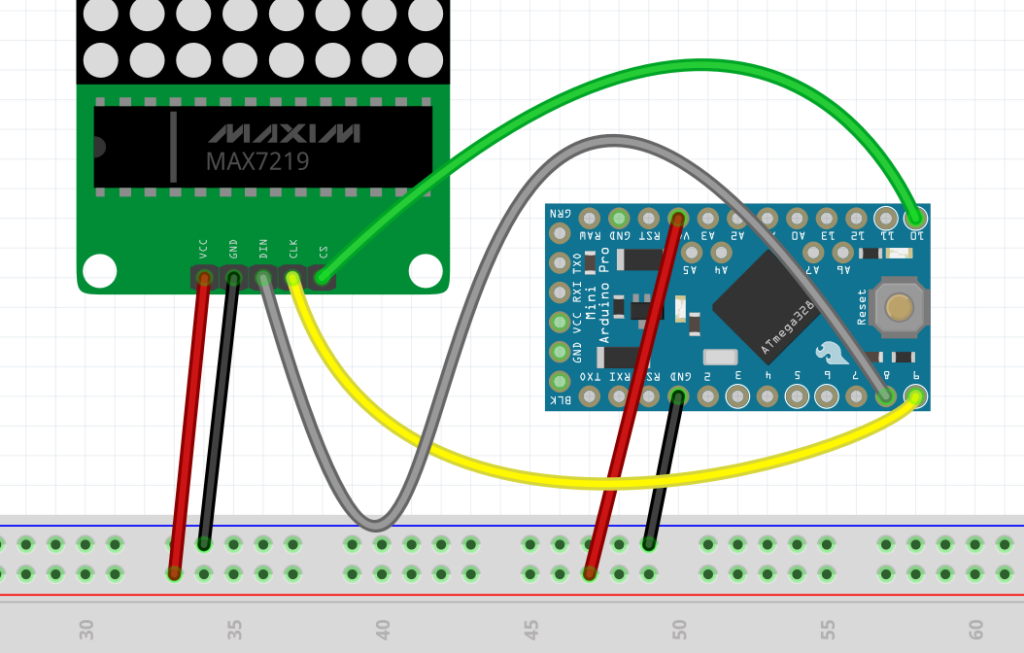
FIrst off, we drop a testing code for the DotMatrix
#define PIN_DIN 8 #define PIN_CLK 9 #define PIN_CS 10 #define NUM_DEVICES 1 #include <LedControl.h> LedControl lc = LedControl(PIN_DIN, PIN_CLK, PIN_CS, NUM_DEVICES); void setup() { lc.shutdown(0, false); // intensity level (0..15) lc.setIntensity(0, 15); lc.clearDisplay(0); } void loop() { int rx = (int)(random(8)); int ry = (int)(random(8)); if (random(100) > 50) { lc.setLed(0, rx, ry, true); } else { lc.setLed(0, rx, ry, false); } }
processing code
import processing.serial.*; Serial myPort; // Create object from Serial class boolean[] ledState = new boolean[64]; // Array to store LED states int cellSize = 50; void setup() { size(400, 400); String portName = Serial.list()[0]; // Adjust the port index as needed myPort = new Serial(this, portName, 9600); // Initialize all LEDs as off for (int i = 0; i < ledState.length; i++) { ledState[i] = false; } // Send special command to reset Arduino state buffer resetArduinoState(); } void draw() { background(0); // Draw LED grid for (int row = 0; row < 8; row++) { for (int col = 0; col < 8; col++) { int id = row * 8 + col; // Determine fill color based on LED state fill(ledState[id] ? color(255, 0, 0) : color(20)); ellipse(col * cellSize + cellSize / 2, row * cellSize + cellSize / 2, cellSize * 0.8, cellSize * 0.8); } } // Periodically send special command to keep Arduino state in sync if (frameCount % 300 == 0) { // Adjust the period (300 frames = approx. 5 seconds at 60 fps) // resetArduinoState(); } } void mousePressed() { // Calculate which LED was clicked and send its ID over serial int row = mouseY / cellSize; int col = mouseX / cellSize; int id = row * 8 + col; myPort.write(id); // Send LED ID (0 to 63) over serial // Toggle local state and update visualization ledState[id] = !ledState[id]; } void resetArduinoState() { myPort.write(255); // Send special command (255) over serial to reset Arduino state println("reset ARDUINO"); }
#include <LedControl.h> #define PIN_DIN 8 #define PIN_CLK 9 #define PIN_CS 10 #define NUM_DEVICES 1 LedControl lc = LedControl(PIN_DIN, PIN_CLK, PIN_CS, NUM_DEVICES); bool ledState[64] = {false}; // State buffer for 64 LEDs void setup() { Serial.begin(9600); lc.shutdown(0, false); lc.setIntensity(0, 15); lc.clearDisplay(0); // Initialize all LEDs as off for (int i = 0; i < 64; i++) { ledState[i] = false; } } void updateLED(int id, bool state) { // Map LED index to row and column for 90 degrees clockwise rotation int row = id % 8; // Row is now column index int col = 7 - (id / 8); // Column is now mirrored row index lc.setLed(0, row, col, state); ledState[id] = state; // Update state buffer } void loop() { // Check if special command (255) is received to reset LED state buffer if (Serial.available() > 0) { int specialCommand = Serial.read(); if (specialCommand == 255) { // Reset LED state buffer for (int i = 0; i < 64; i++) { updateLED(i, false); // Turn off all LEDs } } else { // Toggle LED state based on received LED ID int id = specialCommand; bool state = !ledState[id]; // Toggle state updateLED(id, state); } } }
ANIMATION 🙂
import processing.serial.*; import java.util.ArrayList; Serial myPort; // Create object from Serial class boolean[] ledState = new boolean[64]; // Array to store LED states int cellSize = 50; ArrayList<Integer> recordedCommands = new ArrayList<Integer>(); // ArrayList to store recorded LED IDs ArrayList<Long> timestamps = new ArrayList<Long>(); // ArrayList to store timestamps boolean recording = false; // Flag to indicate recording state long lastRecordTime = 0; // Last recorded timestamp boolean playingBack = false; // Flag to indicate playback state int playbackIndex = 0; // Index for current playback command void setup() { size(400, 400); String portName = Serial.list()[0]; // Adjust the port index as needed myPort = new Serial(this, portName, 9600); // Initialize all LEDs as off for (int i = 0; i < ledState.length; i++) { ledState[i] = false; } // Send special command to reset Arduino state buffer resetArduinoState(); } void draw() { background(0); // Draw LED grid for (int row = 0; row < 8; row++) { for (int col = 0; col < 8; col++) { int id = row * 8 + col; // Determine fill color based on LED state fill(ledState[id] ? color(255, 0, 0) : color(20)); ellipse(col * cellSize + cellSize / 2, row * cellSize + cellSize / 2, cellSize * 0.8, cellSize * 0.8); } } // Check if playback is active if (playingBack) { sendPlaybackCommand(); // Send the next playback command } } void keyPressed() { if (key == ' ') { if (!recording && !playingBack) { startRecording(); } else if (recording) { stopRecording(); playbackCommands(); } else if (playingBack) { stopPlayback(); resetArduinoState(); } } } void mousePressed() { // Check if the mouse is inside the recording button area if (mouseX > 50 && mouseX < 150 && mouseY > 420 && mouseY < 470) { if (!recording && !playingBack) { startRecording(); } else if (recording) { stopRecording(); playbackCommands(); } else if (playingBack) { stopPlayback(); resetArduinoState(); } } // Check if the mouse is inside the clear button area if (mouseX > 250 && mouseX < 350 && mouseY > 420 && mouseY < 470) { clearRecording(); } // If recording, record which LED was clicked if (recording && mouseX >= 0 && mouseX < width && mouseY >= 0 && mouseY < height) { int row = mouseY / cellSize; int col = mouseX / cellSize; int id = row * 8 + col; recordedCommands.add(id); // Record LED ID timestamps.add(millis() - lastRecordTime); // Record time since last command lastRecordTime = millis(); // Update last record time // Toggle local state and update visualization ledState[id] = !ledState[id]; } } void startRecording() { recording = true; recordedCommands.clear(); // Clear any previous recorded commands timestamps.clear(); // Clear any previous recorded timestamps lastRecordTime = millis(); // Initialize last record time // Initialize all LEDs as off for (int i = 0; i < ledState.length; i++) { ledState[i] = false; } } void stopRecording() { recording = false; } void playbackCommands() { if (recordedCommands.size() > 0) { playingBack = true; playbackIndex = 0; } } void sendPlaybackCommand() { if (playingBack) { if (playbackIndex < recordedCommands.size()) { int id = recordedCommands.get(playbackIndex); myPort.write(id); // Send LED ID (0 to 63) over serial delay(int(timestamps.get(playbackIndex))); // Wait for the recorded time delay playbackIndex++; } else { // End of playback, loop infinitely playbackIndex = 0; } } } void stopPlayback() { playingBack = false; } void clearRecording() { recordedCommands.clear(); // Clear recorded commands timestamps.clear(); // Clear timestamps for (int i = 0; i < ledState.length; i++) { ledState[i] = false; // Clear LED states } } void resetArduinoState() { myPort.write(255); // Send special command (255) over serial to reset Arduino state }
// ARDUINO COE MIT BEEPER auf pin3
#include <LedControl.h> #define PIN_DIN 8 #define PIN_CLK 9 #define PIN_CS 10 #define NUM_DEVICES 1 LedControl lc = LedControl(PIN_DIN, PIN_CLK, PIN_CS, NUM_DEVICES); bool ledState[64] = {false}; // State buffer for 64 LEDs void setup() { Serial.begin(9600); lc.shutdown(0, false); lc.setIntensity(0, 15); lc.clearDisplay(0); // Initialize all LEDs as off for (int i = 0; i < 64; i++) { ledState[i] = false; } } void updateLED(int id, bool state) { // Map LED index to row and column for 90 degrees clockwise rotation int row = id % 8; // Row is now column index int col = 7 - (id / 8); // Column is now mirrored row index lc.setLed(0, row, col, state); ledState[id] = state; // Update state buffer } void loop() { // Check if special command (255) is received to reset LED state buffer if (Serial.available() > 0) { int specialCommand = Serial.read(); if (specialCommand == 255) { // Reset LED state buffer for (int i = 0; i < 64; i++) { updateLED(i, false); // Turn off all LEDs } } else { // Toggle LED state based on received LED ID int id = specialCommand; bool state = !ledState[id]; // Toggle state updateLED(id, state); for(int i=0;i<5;i++){ tone(3,40+i*500 + id * 100); delay(30); } noTone(3); } } }