infinite room setup
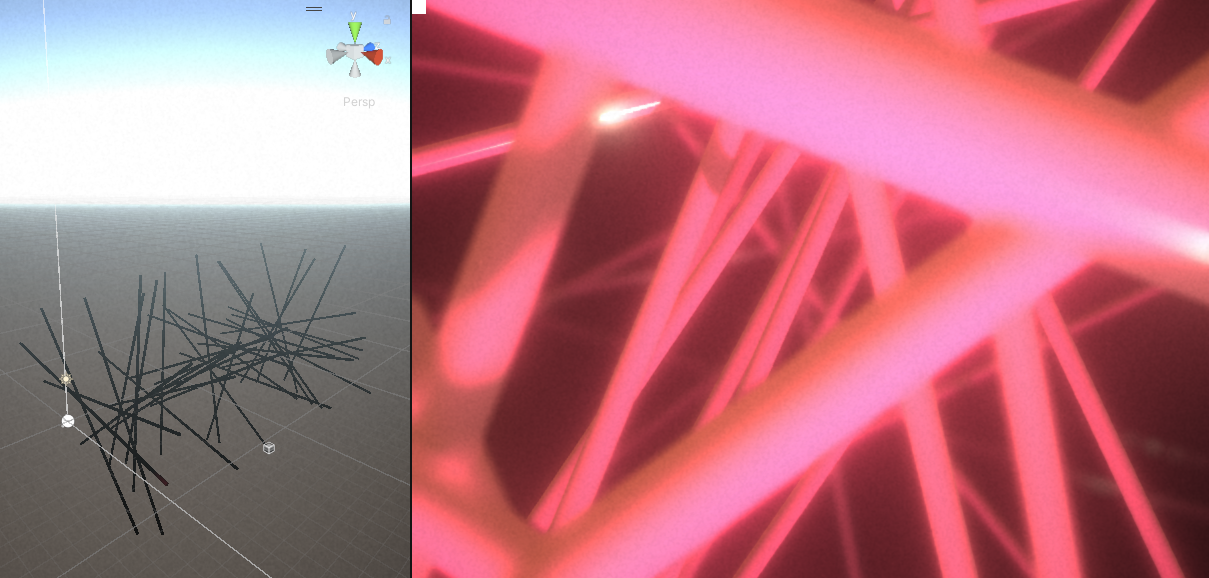
infinite_room_setup – unity package [ DOWNLOAD ]
gaze control basics
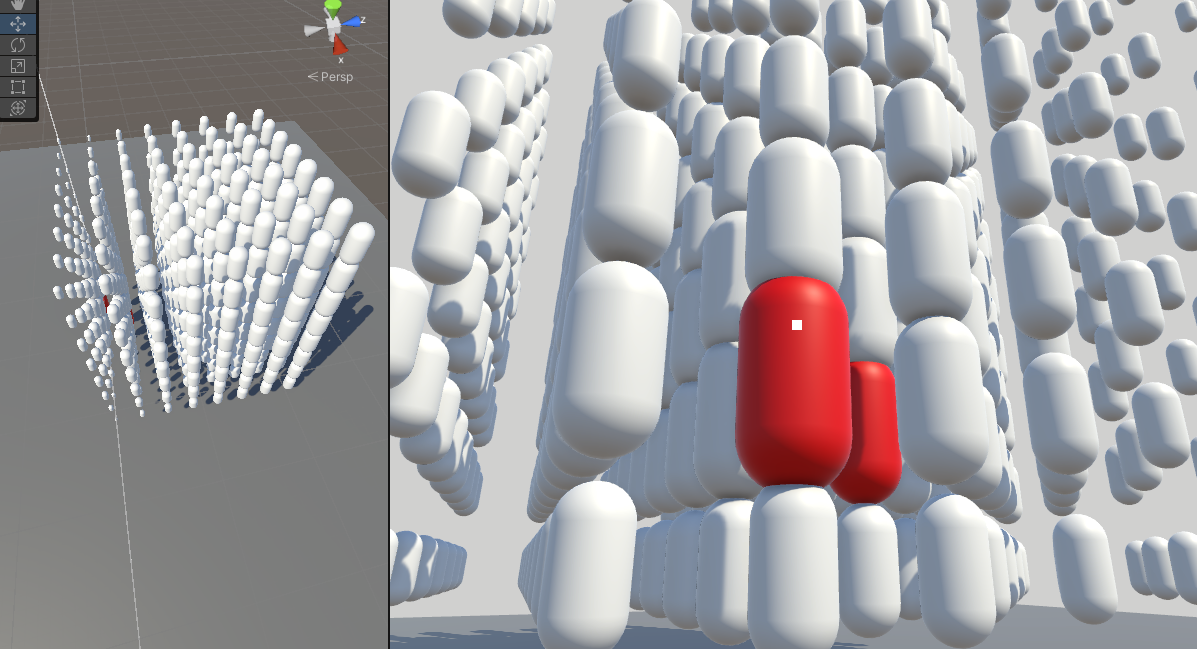
gaze_setup Unity package [DOWNLOAD]
using UnityEngine; public class GazeTrigger : MonoBehaviour { public Camera gazeCamera; // Assign the camera you want to use for raycasting public float gazeDuration = 5f; // Duration required to trigger the gaze private GameObject gazedObject; private float gazeTimer; public Material redMaterial; // Assign the red material in the inspector void Update() { // Create a ray from the camera through the center of the screen Ray ray = gazeCamera.ScreenPointToRay(new Vector3(Screen.width / 2f, Screen.height / 2f, 0f)); RaycastHit hit; // Perform the raycast if (Physics.Raycast(ray, out hit)) { if( hit.collider.gameObject.tag != "tracked_obj" ){return;} // Check if the hit object is different from the previously gazed object if (hit.collider.gameObject != gazedObject) { // Reset the gaze timer if the hit object changes gazedObject = hit.collider.gameObject; gazeTimer = 0f; } else { // Increment the gaze timer if the hit object remains the same gazeTimer += Time.deltaTime; if (gazeTimer >= gazeDuration) { // Trigger the specific function when gaze duration is reached OnGazeTriggered(gazedObject); } } } else { // Reset the gazed object and timer if the raycast doesn't hit anything gazedObject = null; gazeTimer = 0f; } } void OnGazeTriggered(GameObject gazedObject) { Debug.Log("Gaze triggered on: " + gazedObject.name); // Perform your desired action here // Change the material color to red using MaterialPropertyBlock Renderer renderer = gazedObject.GetComponent<Renderer>(); if (renderer != null) { MaterialPropertyBlock materialBlock = new MaterialPropertyBlock(); materialBlock.SetColor("_BaseColor", Color.red); // Assuming you're using "_BaseColor" property in your URP shader renderer.SetPropertyBlock(materialBlock); } } }
using System.Collections; using System.Collections.Generic; using UnityEngine; public class grid_maker : MonoBehaviour { public GameObject go; public float bit_abs; // Start is called before the first frame update void Start() { int cnt = 0; for(int i=0;i<8;i++){ for(int j=0;j<8;j++){ for(int k=0;k<8;k++){ GameObject ngo = Instantiate(go); ngo.name = "zepfi_" + cnt; cnt++; Vector3 gpos = new Vector3(i*bit_abs,j*bit_abs,k*bit_abs); ngo.transform.position = gpos; } } } } }
using System.Collections; using System.Collections.Generic; using UnityEngine; public class aBLOB : MonoBehaviour { public Camera gaze_cam; // Start is called before the first frame update void Start() { gaze_cam = GameObject.Find("gaze_camera").GetComponent<Camera>();; } // Update is called once per frame void Update() { gazeUpdate(); } void gazeUpdate(){ // Get the center of the screen in pixel coordinates Vector2 screenCenter = new Vector2(Screen.width / 2f, Screen.height / 2f); // Convert the world position of the object to screen space Vector3 screenPoint = gaze_cam.WorldToScreenPoint(this.transform.position); // Calculate the distance between the screen center and the object's screen position float distanceToCenter = Vector2.Distance(screenCenter, new Vector2(screenPoint.x, screenPoint.y)); if(distanceToCenter<600f){ float s = 0.3f + ( 601f-distanceToCenter )* .003f; this.transform.localScale = new Vector3(s,s,s); } } }
dynamic room made from objects with follow behaviour
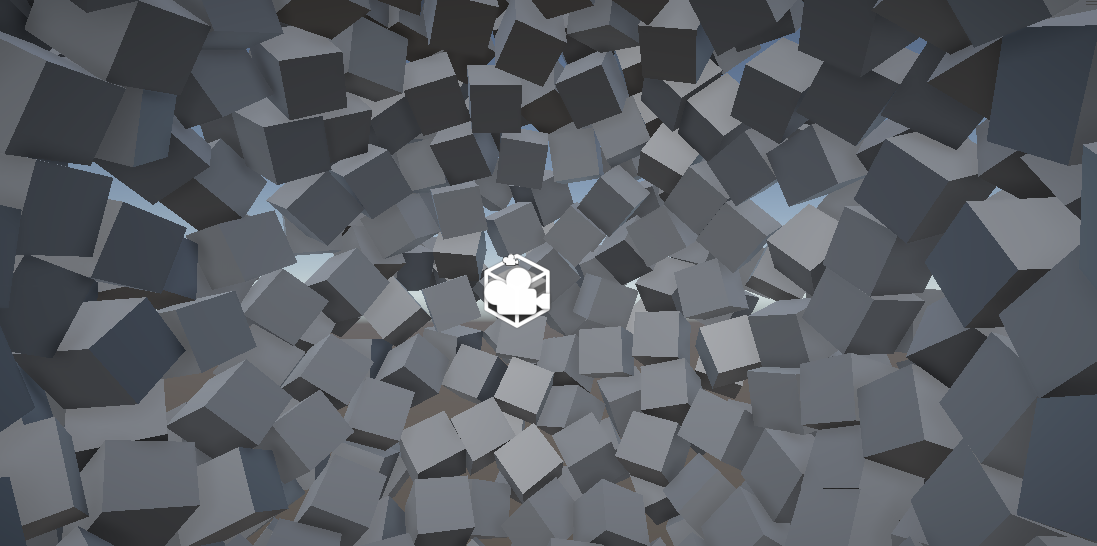
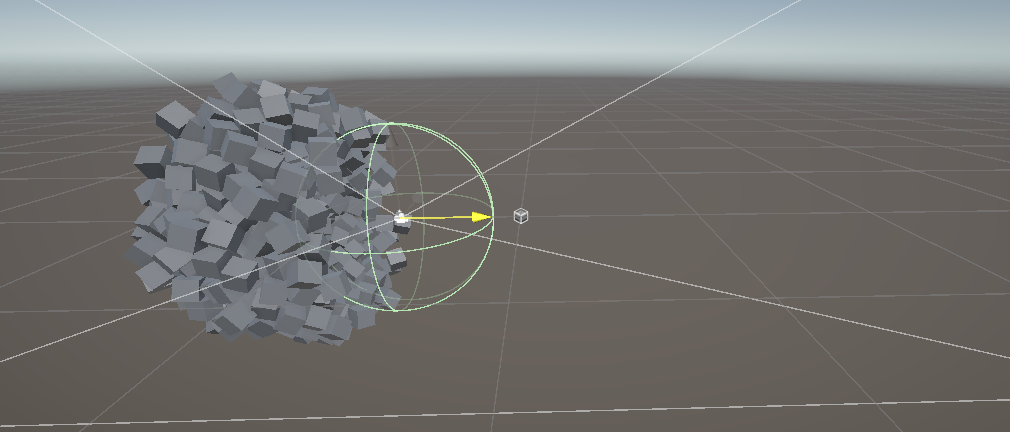
Download all scripts and basic setup for Unity URP: magnetic_boxes_basic [UNITY PACKAGE]
using System.Collections; using System.Collections.Generic; using UnityEngine; public class aCUBE : MonoBehaviour { public Rigidbody rb; public GameObject the_player_rig; void Start() { the_player_rig = GameObject.Find("MAIN_RIG"); } // Update is called once per frame void Update() { // calculate the differential vector to the main player Vector3 diff = this.transform.position - the_player_rig.transform.position; //new Vector3( 0,0,3f); // add a constant calculated force to parent cube - NOT normalized! rb.AddForce( -diff * .2f ); } }
using System.Collections; using System.Collections.Generic; using UnityEngine; public class main_controller : MonoBehaviour { public GameObject go; void Start() { create_boxes(); } void create_boxes(){ // create 510 instances of a premade prefab at random position for( int i =0; i<511; i++){ GameObject ngo = Instantiate(go); Vector3 rpos = Vector3.zero; rpos.x = Random.Range( -4f,4f ); rpos.y = Random.Range( -4f,4f ); rpos.z = Random.Range( -4f,4f ); ngo.transform.position = rpos; } } }